Dependent Density Regression with PyMC3
My last post showed how to use Dirichlet processes and pymc3
to perform Bayesian nonparametric density estimation. This post expands on the previous one, illustrating dependent density regression with pymc3
.
Just as Dirichlet process mixtures can be thought of as infinite mixture models that select the number of active components as part of inference, dependent density regression can be thought of as infinite mixtures of experts that select the active experts as part of inference. Their flexibility and modularity make them powerful tools for performing nonparametric Bayesian Data analysis.
%matplotlib inline
from IPython.display import HTML
from matplotlib import animation as ani, pyplot as plt
import numpy as np
import pandas as pd
import pymc3 as pm
import seaborn as sns
from theano import shared, tensor as tt
'animation', writer='avconv')
plt.rc(*_ = sns.color_palette() blue,
= 972915 # from random.org; for reproducibility
SEED np.random.seed(SEED)
Throughout this post, we will use the LIDAR data set from Larry Wasserman’s excellent book, All of Nonparametric Statistics. We standardize the data set to improve the rate of convergence of our samples.
= 'http://www.stat.cmu.edu/~larry/all-of-nonpar/=data/lidar.dat'
DATA_URI
def standardize(x):
return (x - x.mean()) / x.std()
= (pd.read_csv(DATA_URI, sep=' *', engine='python')
df =lambda df: standardize(df.range),
.assign(std_range=lambda df: standardize(df.logratio))) std_logratio
df.head()
range | logratio | std_logratio | std_range | |
---|---|---|---|---|
0 | 390 | -0.050356 | 0.852467 | -1.717725 |
1 | 391 | -0.060097 | 0.817981 | -1.707299 |
2 | 393 | -0.041901 | 0.882398 | -1.686447 |
3 | 394 | -0.050985 | 0.850240 | -1.676020 |
4 | 396 | -0.059913 | 0.818631 | -1.655168 |
We plot the LIDAR data below.
= plt.subplots(figsize=(8, 6))
fig, ax
ax.scatter(df.std_range, df.std_logratio,=blue);
c
;
ax.set_xticklabels([])"Standardized range");
ax.set_xlabel(
;
ax.set_yticklabels([])"Standardized log ratio"); ax.set_ylabel(
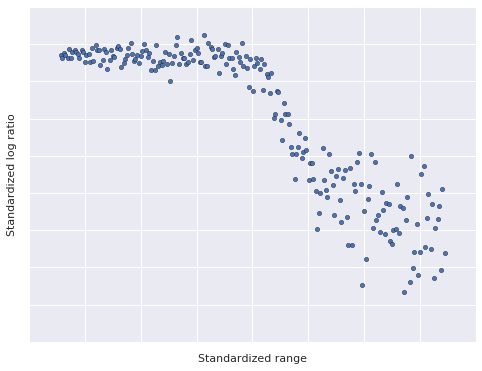
This data set has a two interesting properties that make it useful for illustrating dependent density regression.
- The relationship between range and log ratio is nonlinear, but has locally linear components.
- The observation noise is heteroskedastic; that is, the magnitude of the variance varies with the range.
The intuitive idea behind dependent density regression is to reduce the problem to many (related) density estimates, conditioned on fixed values of the predictors. The following animation illustrates this intuition.
= plt.subplots(ncols=2, figsize=(16, 6))
fig, (scatter_ax, hist_ax)
scatter_ax.scatter(df.std_range, df.std_logratio,=blue, zorder=2);
c
;
scatter_ax.set_xticklabels([])"Standardized range");
scatter_ax.set_xlabel(
;
scatter_ax.set_yticklabels([])"Standardized log ratio");
scatter_ax.set_ylabel(
= np.linspace(df.std_range.min(), df.std_range.max(), 25)
bins
=bins,
hist_ax.hist(df.std_logratio, bins='k', lw=0, alpha=0.25,
color="All data");
label
;
hist_ax.set_xticklabels([])"Standardized log ratio");
hist_ax.set_xlabel(
;
hist_ax.set_yticklabels([])"Frequency");
hist_ax.set_ylabel(
=2);
hist_ax.legend(loc
= np.linspace(1.05 * df.std_range.min(), 1.05 * df.std_range.max(), 15)
endpoints
= []
frame_artists
for low, high in zip(endpoints[:-1], endpoints[2:]):
= scatter_ax.axvspan(low, high,
interval ='k', alpha=0.5, lw=0, zorder=1);
color*_, bars = hist_ax.hist(df[df.std_range.between(low, high)].std_logratio,
=bins,
bins='k', lw=0, alpha=0.5);
color
+ tuple(bars))
frame_artists.append((interval,)
= ani.ArtistAnimation(fig, frame_artists,
animation =500, repeat_delay=3000, blit=True)
interval; # prevent the intermediate figure from showing plt.close()
HTML(animation.to_html5_video())
As we slice the data with a window sliding along the x-axis in the left plot, the empirical distribution of the y-values of the points in the window varies in the right plot. An important aspect of this approach is that the density estimates that correspond to close values of the predictor are similar.
In the previous post, we saw that a Dirichlet process estimates a probability density as a mixture model with infinitely many components. In the case of normal component distributions,
\[ y \sim \sum_{i = 1}^{\infty} w_i \cdot N(\mu_i, \tau_i^{-1}), \]
where the mixture weights, \(w_1, w_2, \ldots\), are generated by a stick-breaking process.
Dependent density regression generalizes this representation of the Dirichlet process mixture model by allowing the mixture weights and component means to vary conditioned on the value of the predictor, \(x\). That is,
\[ y\ |\ x \sim \sum_{i = 1}^{\infty} w_i\ |\ x \cdot N(\mu_i\ |\ x, \tau_i^{-1}). \]
In this post, we will follow Chapter 23 of Bayesian Data Analysis and use a probit stick-breaking process to determine the conditional mixture weights, \(w_i\ |\ x\). The probit stick-breaking process starts by defining
\[ v_i\ |\ x = \Phi(\alpha_i + \beta_i x), \]
where \(\Phi\) is the cumulative distribution function of the standard normal distribution. We then obtain \(w_i\ |\ x\) by applying the stick breaking process to \(v_i\ |\ x\). That is,
\[ w_i\ |\ x = v_i\ |\ x \cdot \prod_{j = 1}^{i - 1} (1 - v_j\ |\ x). \]
For the LIDAR data set, we use independent normal priors \(\alpha_i \sim N(0, 5^2)\) and \(\beta_i \sim N(0, 5^2)\). We now express this this model for the conditional mixture weights using pymc3
.
def norm_cdf(z):
return 0.5 * (1 + tt.erf(z / np.sqrt(2)))
def stick_breaking(v):
return v * tt.concatenate([tt.ones_like(v[:, :1]),
1 - v, axis=1)[:, :-1]],
tt.extra_ops.cumprod(=1) axis
= df.shape
N, _ = 20
K
= df.std_range.values[:, np.newaxis]
std_range = df.std_logratio.values[:, np.newaxis]
std_logratio
= shared(std_range, broadcastable=(False, True))
x_lidar
with pm.Model() as model:
= pm.Normal('alpha', 0., 5., shape=K)
alpha = pm.Normal('beta', 0., 5., shape=K)
beta = norm_cdf(alpha + beta * x_lidar)
v = pm.Deterministic('w', stick_breaking(v)) w
We have defined x_lidar
as a theano
shared
variable in order to use pymc3
’s posterior prediction capabilities later.
While the dependent density regression model theoretically has infinitely many components, we must truncate the model to finitely many components (in this case, twenty) in order to express it using pymc3
. After sampling from the model, we will verify that truncation did not unduly influence our results.
Since the LIDAR data seems to have several linear components, we use the linear models
\[ \begin{align*} \mu_i\ |\ x & \sim \gamma_i + \delta_i x \\ \gamma_i & \sim N(0, 10^2) \\ \delta_i & \sim N(0, 10^2) \end{align*} \]
for the conditional component means.
with model:
= pm.Normal('gamma', 0., 10., shape=K)
gamma = pm.Normal('delta', 0., 10., shape=K)
delta = pm.Deterministic('mu', gamma + delta * x_lidar) mu
Finally, we place the prior \(\tau_i \sim \textrm{Gamma}(1, 1)\) on the component precisions.
with model:
= pm.Gamma('tau', 1., 1., shape=K)
tau = pm.NormalMixture('obs', w, mu, tau=tau, observed=std_logratio) obs
We now draw sample from the dependent density regression model.
= 20000
SAMPLES = 10000
BURN = 10
THIN
with model:
= pm.Metropolis()
step = pm.sample(SAMPLES, step, random_seed=SEED)
trace_
= trace_[BURN::THIN] trace
100%|██████████| 20000/20000 [01:30<00:00, 204.48it/s]
To verify that truncation did not unduly influence our results, we plot the largest posterior expected mixture weight for each component. (In this model, each point has a mixture weight for each component, so we plot the maximum mixture weight for each component across all data points in order to judge if the component exerts any influence on the posterior.)
= plt.subplots(figsize=(8, 6))
fig, ax
+ 1 - 0.4,
ax.bar(np.arange(K) 'w'].mean(axis=0).max(axis=0));
trace[
1 - 0.5, K + 0.5);
ax.set_xlim('Mixture component');
ax.set_xlabel(
'Largest posterior expected\nmixture weight'); ax.set_ylabel(
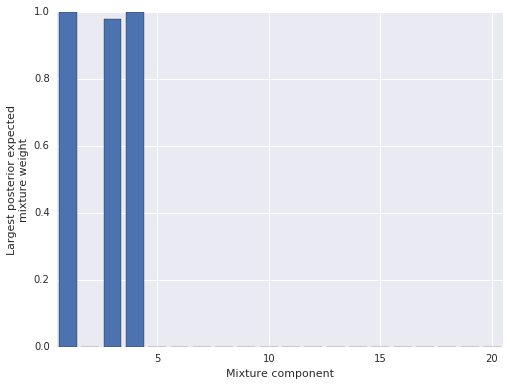
Since only three mixture components have appreciable posterior expected weight for any data point, we can be fairly certain that truncation did not unduly influence our results. (If most components had appreciable posterior expected weight, truncation may have influenced the results, and we would have increased the number of components and sampled again.)
Visually, it is reasonable that the LIDAR data has three linear components, so these posterior expected weights seem to have identified the structure of the data well. We now sample from the posterior predictive distribution to get a better understand the model’s performance.
= 5000
PP_SAMPLES
= np.linspace(std_range.min() - 0.05, std_range.max() + 0.05, 100)
lidar_pp_x
x_lidar.set_value(lidar_pp_x[:, np.newaxis])
with model:
= pm.sample_ppc(trace, PP_SAMPLES, random_seed=SEED) pp_trace
100%|██████████| 5000/5000 [01:18<00:00, 66.54it/s]
Below we plot the posterior expected value and the 95% posterior credible interval.
= plt.subplots()
fig, ax
ax.scatter(df.std_range, df.std_logratio,=blue, zorder=10,
c=None);
label
= np.percentile(pp_trace['obs'], [2.5, 97.5], axis=0)
low, high
ax.fill_between(lidar_pp_x, low, high,='k', alpha=0.35, zorder=5,
color='95% posterior credible interval');
label
'obs'].mean(axis=0),
ax.plot(lidar_pp_x, pp_trace[='k', zorder=6,
c='Posterior expected value');
label
;
ax.set_xticklabels([])'Standardized range');
ax.set_xlabel(
;
ax.set_yticklabels([])'Standardized log ratio');
ax.set_ylabel(
=1);
ax.legend(loc'LIDAR Data'); ax.set_title(
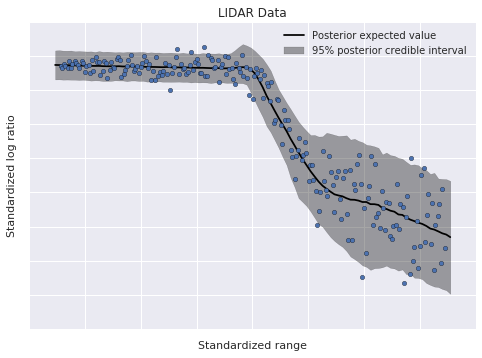
The model has fit the linear components of the data well, and also accomodated its heteroskedasticity. This flexibility, along with the ability to modularly specify the conditional mixture weights and conditional component densities, makes dependent density regression an extremely useful nonparametric Bayesian model.
To learn more about depdendent density regression and related models, consult Bayesian Data Analysis, Bayesian Nonparametric Data Analysis, or Bayesian Nonparametrics.
This post is available as a Jupyter notebook here.